Today I was asked to discuss the SOLID principles. But how to explain it to beginners? How to explain it to non-tech people? OK, let’s figure it out together.
When I try to explain something to someone, I am usually verbose. I tend to deep dive into HOW or WHY things became that way instead of straightly explaining WHAT is that thing. I’m sorry if I bored you doing that once (maybe more than twice 😊) in the past. That’s how I learn things… But if you’re in a rush to understand right now, I’ll try to do things backward.
I’ll try to use easy-to-understand language, but this is not supposed to be an article for beginners. If you’re running for a Junior position and the company asks you to explain this, run away from them! Your code needs to smell like sewage before you understand this… Whenever you look at your lines and think “It should be a way to make it better and avoid rewriting that much”, then you’re ready. Maybe… 😁
What is SOLID?
In software programming, SOLID is a mnemonic acronym for five design principles intended to make object-oriented designs more understandable, flexible, and maintainable.
Wikipedia
Now you know WHAT it is. Happy? Probably not, right? So let’s move on.
The acronym
Since it’s an acronym, each letter means a thing. A Principle. They are:
- S = Single Responsibility Principle or SLP
- O = Open-Closed Principle or SRP
- L = Liskov Substitution Principle or SRP
- I = Interface Segregation Principle or IRP
- D = Dependency Inversion Principle or DIP
🎉Congratulations! You have a lot of knowledge now! 🎓
But you’re not happy, probably… I know how you feel. It’s never enough when we talk about learning… We ALWAYS need more! 🤑
So, let’s strip each letter and dive into each of the five principles. I’ll first give some summary and deep dive into them after, OK? And for after, I mean in another article.
Single Responsibility Principle
The SRP stands for the basics: you should never, I said NEVER, create a class, method, or even a mere variable that has more than one responsibility. Simple, right?
It means that your method with more than 100 lines is doing way more than it should! 💩 I will not waste your time trying to convince you that. Just get your code and check it out.
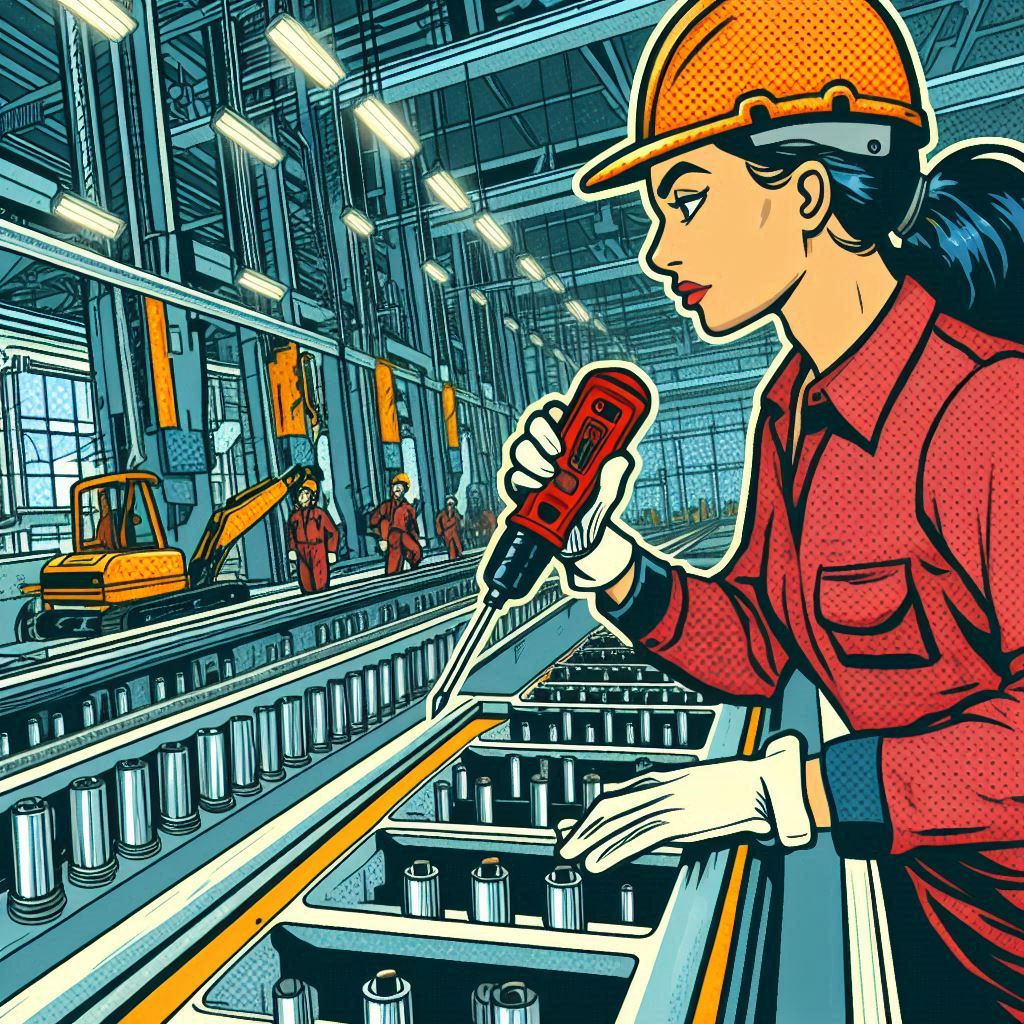
Open-Closed Principle
Here is where people start to get confused and fight each other trying to convince others they have the absolute truth.
The OCP says:
A Module should be OPEN for extension but CLOSED for modification.
But, Anderson… How can I extend without changing? 🤔
It’s a really good question! And the answer is simple: abstraction.
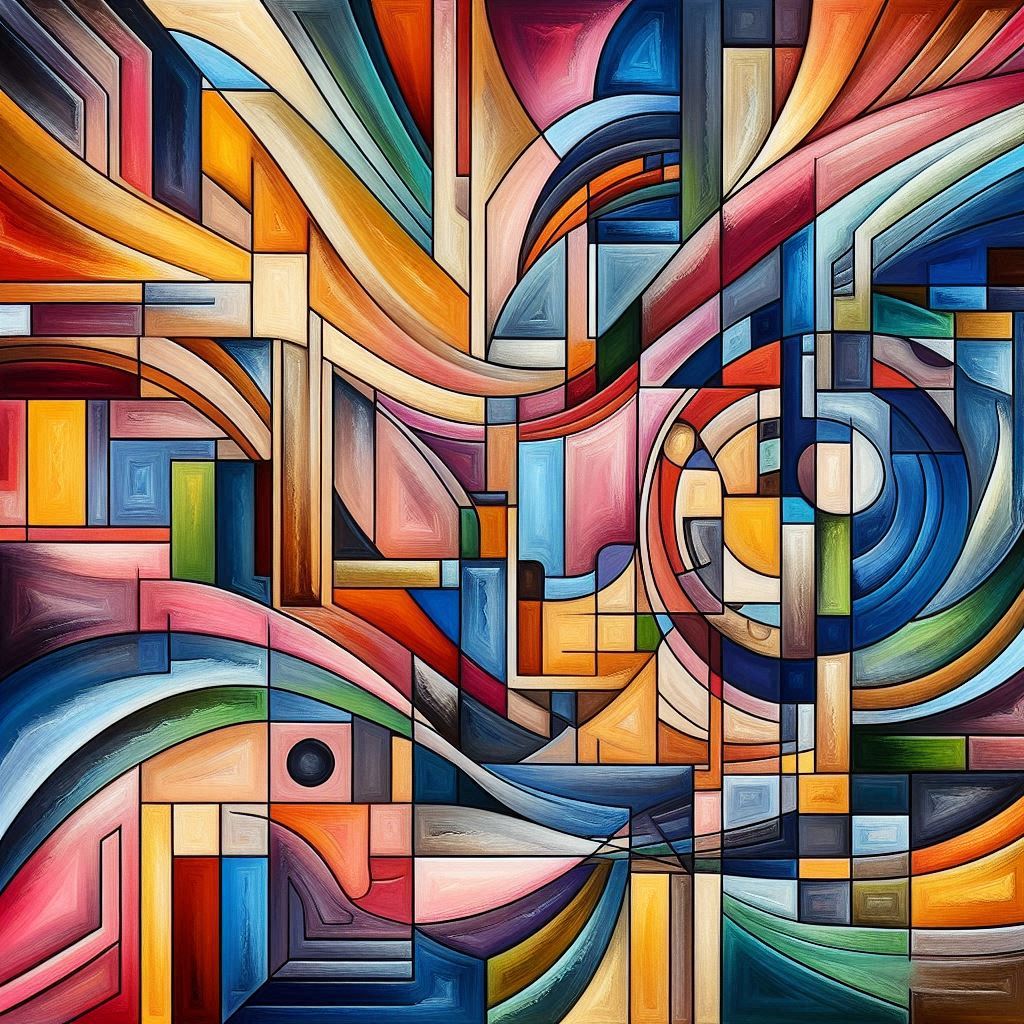
Liskov Substitution Principle
Are you OK? 🤯
To keep LSP straight: if you unplug your wall charger and plug a blender on the same outlet, it needs to work.
For the power outlet, it doesn’t matter what is using the electricity. The only important part is the wall outlet and the plug. They need to be compatible. That’s it.
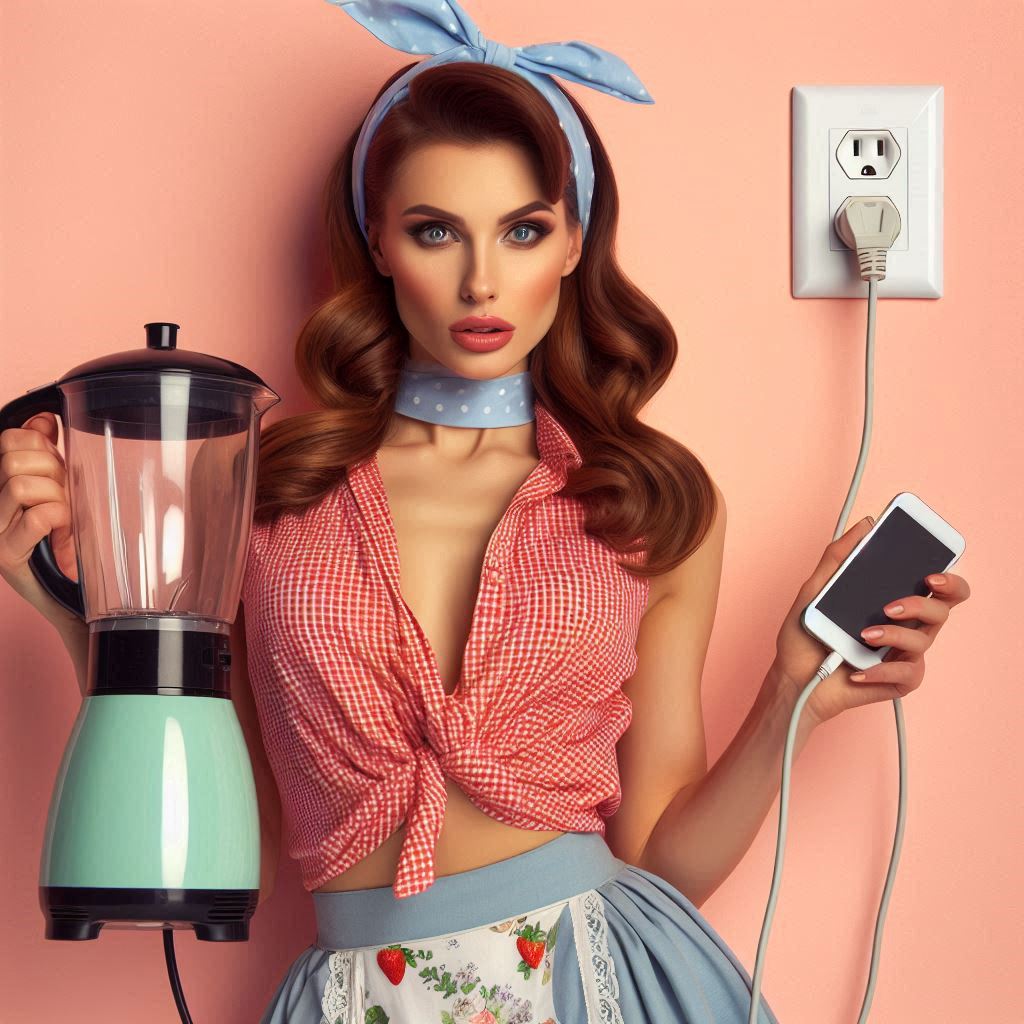
Interface Segregation Principle
Segregation means separation. But here is in a good way, I promise! 🤞
Your interfaces MUST segregate whatever is not 100% necessary to be implemented. If for some reason you need to implement methods that you’re not going to use, you have problems with your Interface.
Rely on ISP means no more MethodNotImplementedException
.
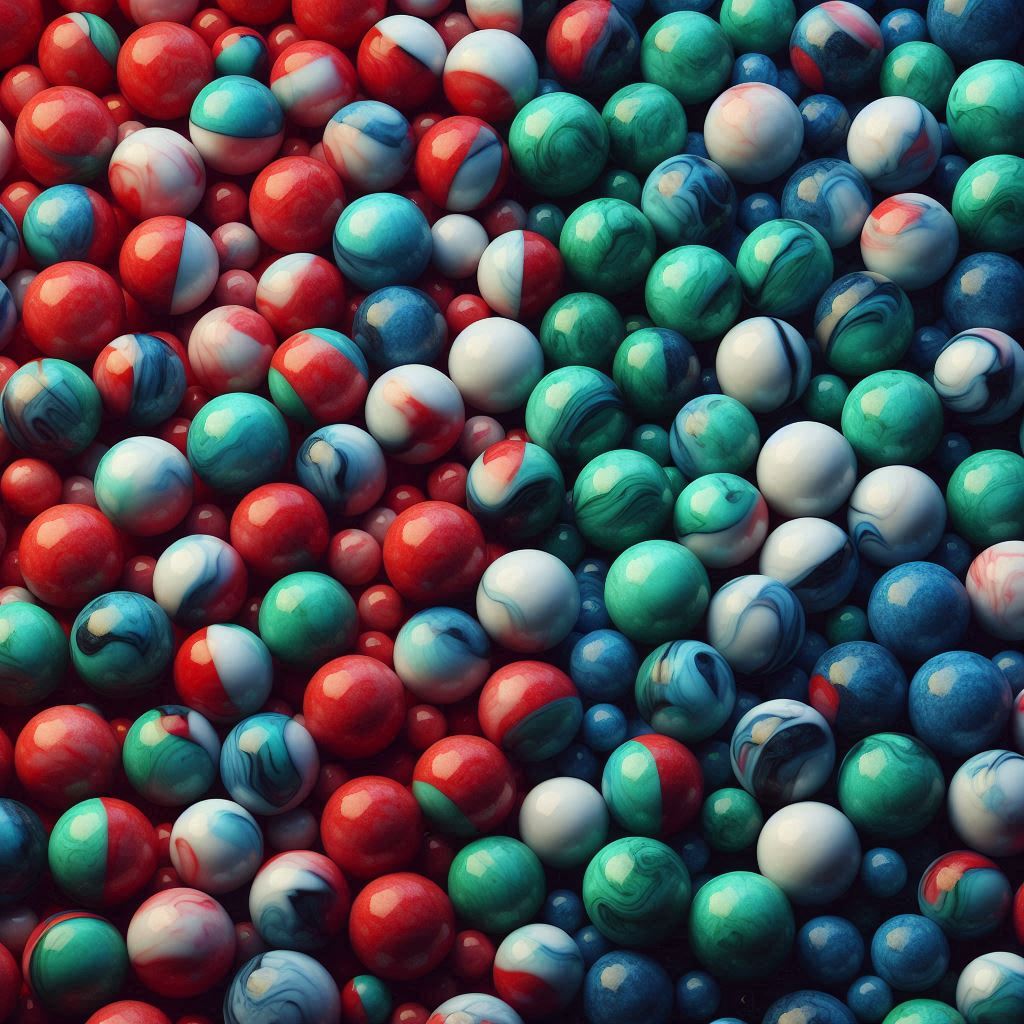
Dependency Inversion Principle
Please, do not confuse it with Dependency Injection. Ok, ok. Dependency Injection IS a Dependency Inversion, indeed. But you can have Dependency Inversion without Dependency Injection.
To what matters for DIP, you only need to know that you should depend on the direction of the abstraction. It means that wherever you instantiate an object that implements your interface, there is where you should set the dependencies. Usually, you will pass the dependencies into the object via the constructor. Sometimes as a method parameter.
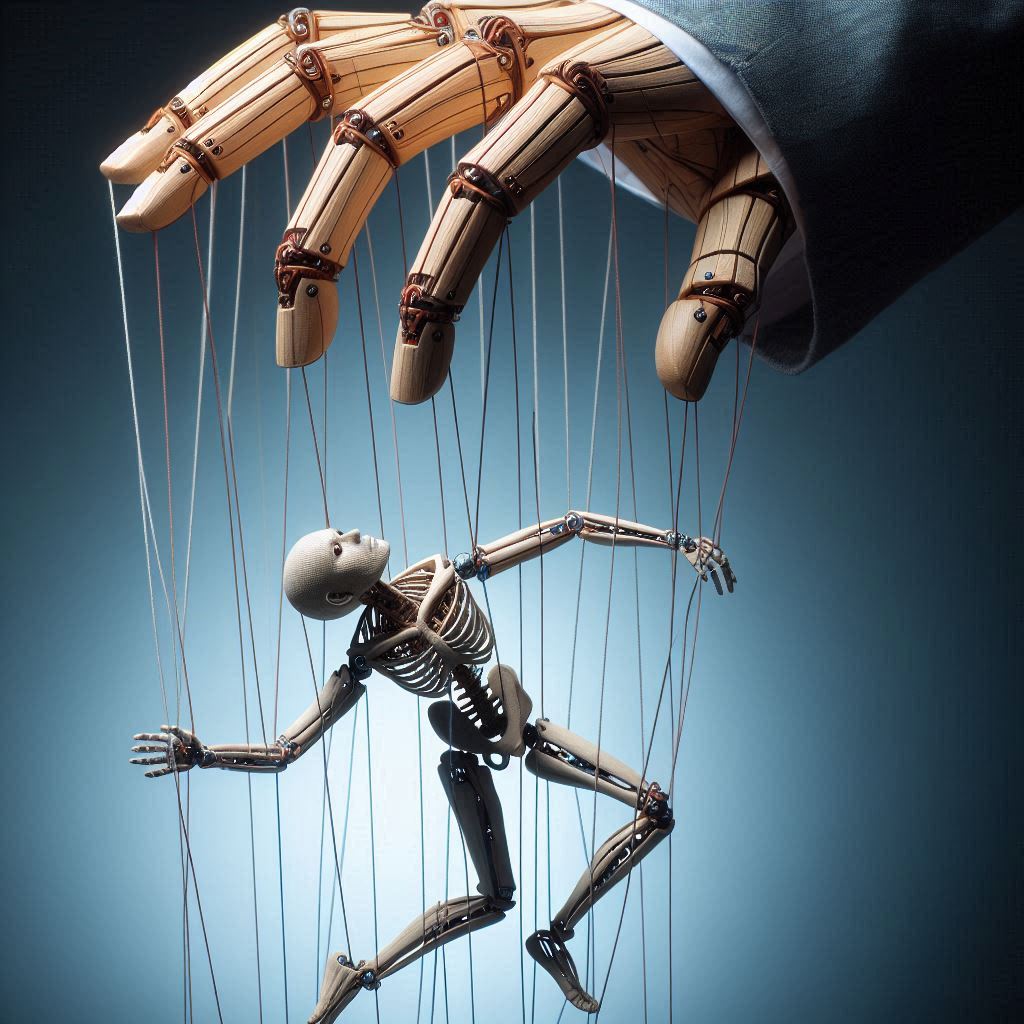
Blah, blah, blah
At this point, you know the basics of the SOLID principles. As I mentioned, this would be an article using easy words, but not intended for beginners.
Without further (technical) explanation, this is pretty much a bunch of blah, blah, blah. That’s why I need to deep dive with some code examples. I’m going to write part 2 of this article and explain how to use the principles in a practical way.
Thanks for reading and see you soon!